This article covers frequently asked data science interview questions at Amazon. Each question is followed by a detailed explanation, relevant code snippets, formulas, and diagrams where needed.
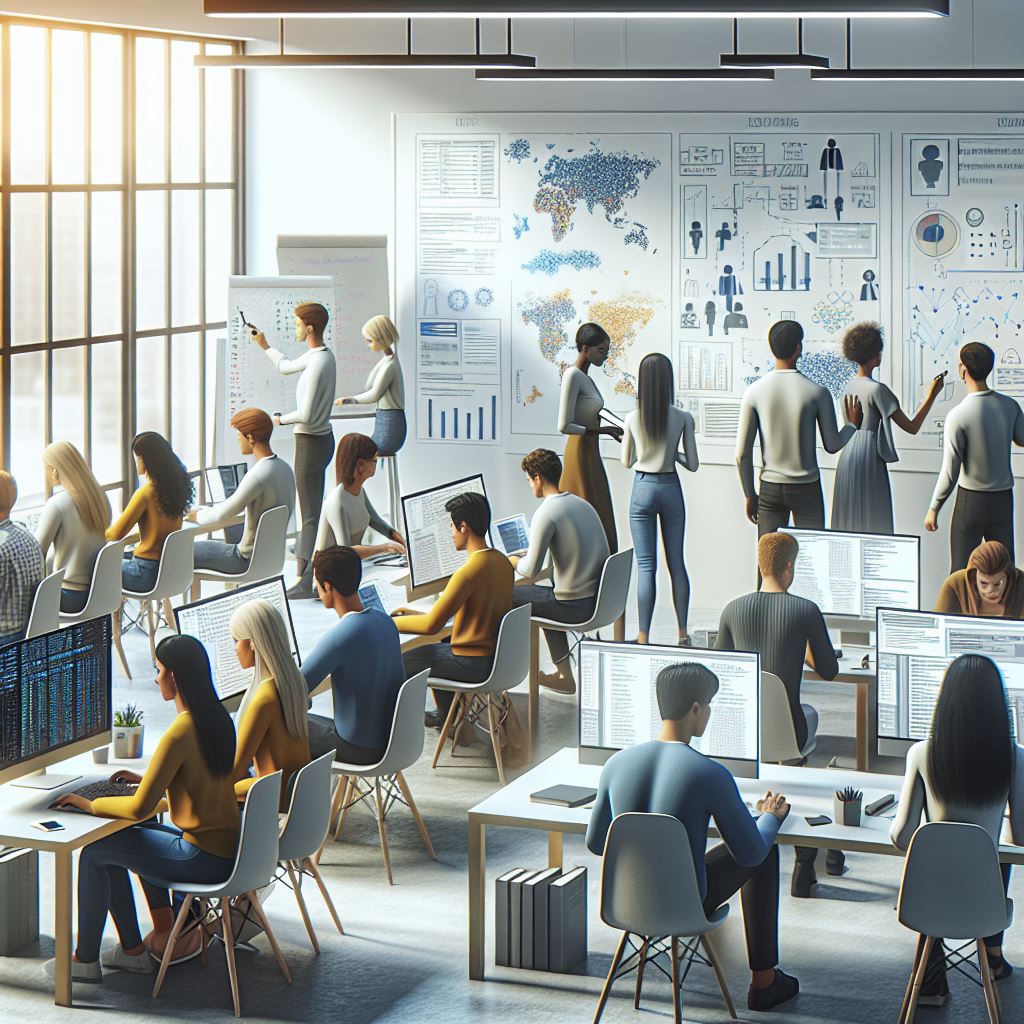
Table of Contents
Data Science Interview Questions and Answers for Amazon
1. What is the difference between supervised, unsupervised, and semi-supervised learning? Provide examples of each.
Supervised Learning: In supervised learning, the model is trained on a labeled dataset, which means that each training example is paired with an output label. The goal is to learn a mapping from inputs to outputs. Examples include:
- Classification: Predicting whether an email is spam or not.
- Regression: Predicting house prices based on features like size, location, etc.
Unsupervised Learning: In unsupervised learning, the model is trained on data without labels. The goal is to find hidden patterns or intrinsic structures in the input data. Examples include:
- Clustering: Grouping customers based on purchasing behavior.
- Dimensionality Reduction: Reducing the number of features in a dataset while retaining important information.
Semi-Supervised Learning: This approach uses a small amount of labeled data and a large amount of unlabeled data. It is useful when labeling data is expensive or time-consuming. Examples include:
- Image Classification: Using a few labeled images and many unlabeled images to improve classification accuracy.
2. Explain overfitting and underfitting in machine learning. How do you prevent them?
Overfitting: Overfitting occurs when a model learns the training data too well, capturing noise and details that do not generalize to new data. It results in high accuracy on training data but poor performance on test data.
Underfitting: Underfitting happens when a model is too simple to capture the underlying patterns in the data, leading to poor performance on both training and test data.
Prevention Techniques:
- Overfitting: Use techniques like cross-validation, regularization (L1, L2), pruning (for decision trees), and dropout (for neural networks).
- Underfitting: Use more complex models, increase the number of features, or reduce regularization.
3. Describe the bias-variance tradeoff. Why is it important in model evaluation?
The bias-variance tradeoff is a fundamental concept in machine learning that describes the tradeoff between two sources of error in a model:
- Bias: Error due to overly simplistic assumptions in the learning algorithm. High bias can cause underfitting.
- Variance: Error due to too much complexity in the learning algorithm. High variance can cause overfitting.
The goal is to find a balance where both bias and variance are minimized to achieve good generalization on unseen data.
4. How would you handle an imbalanced dataset?
Handling imbalanced datasets involves techniques such as:
- Resampling: Oversampling the minority class or undersampling the majority class.
- Synthetic Data Generation: Using techniques like SMOTE (Synthetic Minority Over-sampling Technique) to generate synthetic samples.
- Algorithmic Approaches: Using algorithms that are robust to imbalances, such as decision trees or ensemble methods.
- Evaluation Metrics: Using metrics like precision, recall, F1-score, and ROC-AUC instead of accuracy.
5. What are the key differences between bagging and boosting? When would you use each?
Bagging (Bootstrap Aggregating):
- Purpose: Reduce variance.
- Method: Train multiple models independently on different subsets of the data and average their predictions.
- Example: Random Forest.
Boosting:
- Purpose: Reduce bias.
- Method: Train models sequentially, each new model focusing on the errors of the previous ones.
- Example: AdaBoost, Gradient Boosting.
Usage:
- Bagging: When you want to reduce variance and improve stability.
- Boosting: When you want to reduce bias and improve accuracy.
6. Write code to calculate the F1 score given the confusion matrix.
def calculate_f1_score(confusion_matrix):
TP = confusion_matrix[1, 1]
FP = confusion_matrix[0, 1]
FN = confusion_matrix[1, 0]
precision = TP / (TP + FP)
recall = TP / (TP + FN)
f1_score = 2 * (precision * recall) / (precision + recall)
return f1_score
# Example usage
import numpy as np
confusion_matrix = np.array([[50, 10], [5, 100]])
f1 = calculate_f1_score(confusion_matrix)
print(f"F1 Score: {f1}")
7. How do you optimize a SQL query for large datasets?
Optimization Techniques:
- Indexes: Create indexes on columns used in WHERE, JOIN, and ORDER BY clauses.
- Query Refactoring: Simplify complex queries, avoid subqueries, and use joins efficiently.
- Partitioning: Split large tables into smaller, more manageable pieces.
- Caching: Use caching mechanisms to store frequently accessed data.
- Database Design: Normalize or denormalize tables based on query patterns.
8. Explain the significance of feature scaling. How would you implement it in Python?
Significance: Feature scaling ensures that all features contribute equally to the model’s performance. It is crucial for algorithms that rely on distance metrics, such as k-NN and SVM.
Implementation in Python:
from sklearn.preprocessing import StandardScaler, MinMaxScaler
# Standardization
scaler = StandardScaler()
scaled_data = scaler.fit_transform(data)
# Normalization
scaler = MinMaxScaler()
scaled_data = scaler.fit_transform(data)
9. Describe how to implement k-fold cross-validation in Python and its benefits.
Implementation:
from sklearn.model_selection import KFold, cross_val_score
from sklearn.ensemble import RandomForestClassifier
# Example data and model
X = ...
y = ...
model = RandomForestClassifier()
# k-fold cross-validation
kf = KFold(n_splits=5, shuffle=True, random_state=42)
scores = cross_val_score(model, X, y, cv=kf)
print(f"Cross-validation scores: {scores}")
print(f"Mean score: {scores.mean()}")
Benefits:
- Provides a more reliable estimate of model performance.
- Reduces the risk of overfitting by using different subsets of data for training and validation.
10. What is p-value in hypothesis testing? How do you interpret it?
P-value: The p-value is the probability of obtaining test results at least as extreme as the observed results, assuming the null hypothesis is true. It helps determine the statistical significance of the results.
Interpretation:
- Low p-value (< 0.05): Reject the null hypothesis (evidence against the null hypothesis).
- High p-value (≥ 0.05): Fail to reject the null hypothesis (insufficient evidence against the null hypothesis).
11. Explain the concept of correlation vs. causation. How can you identify causation in a dataset?
Correlation: Measures the strength and direction of a linear relationship between two variables. It does not imply causation.
Causation: Indicates that one event is the result of the occurrence of the other event.
Identifying Causation:
- Randomized Controlled Trials (RCTs): The gold standard for establishing causation.
- Natural Experiments: Observational studies where the assignment of treatment is random.
- Instrumental Variables: Variables that affect the treatment but not the outcome directly.
- Granger Causality: A statistical hypothesis test for determining whether one time series can predict another.
12. What is the curse of dimensionality, and how does it affect machine learning?
Curse of Dimensionality: Refers to the exponential increase in computational complexity and data sparsity as the number of features (dimensions) increases. It affects machine learning by:
- Increasing the risk of overfitting.
- Making distance metrics less meaningful.
- Requiring more data to achieve the same level of performance.
Mitigation Techniques:
- Dimensionality Reduction: Techniques like PCA (Principal Component Analysis) and t-SNE.
- Feature Selection: Selecting the most relevant features using methods like LASSO.
13. How would you deal with missing data in a dataset? Provide specific techniques.
Techniques:
- Deletion: Remove rows or columns with missing values (only if the amount of missing data is small).
- Imputation: Fill in missing values using mean, median, mode, or more sophisticated methods like KNN imputation.
- Prediction Models: Use machine learning models to predict missing values.
- Indicator Variables: Create a binary indicator variable to flag missing values.
14. You are given a dataset with millions of rows. How would you approach exploratory data analysis (EDA) efficiently?
Approach:
- Sampling: Use a representative sample of the data for initial analysis.
- Data Aggregation: Summarize data using group-by operations.
- Visualization: Use efficient visualization libraries like Dask or Vaex for large datasets.
- Parallel Processing: Utilize parallel processing libraries like Dask to handle large datasets.
Sure! Here’s an example of a challenging data science project:
Project: Predicting Customer Churn for a Subscription-Based Service
Problem: The company was facing high customer churn rates, which was impacting revenue. The goal was to predict which customers were likely to churn and take proactive measures to retain them.
Steps Taken:
- Data Collection:
- Gathered data from various sources, including customer interactions, usage patterns, demographics, and transaction history.
- Exploratory Data Analysis (EDA):
- Conducted EDA to understand the data distribution, identify missing values, and detect outliers.
- Visualized data using histograms, box plots, and scatter plots to identify patterns and correlations.
- Data Preprocessing:
- Handled missing values using imputation techniques.
- Performed feature scaling to normalize the data.
- Created new features based on domain knowledge (e.g., average usage per month, number of support tickets).
- Feature Selection:
- Used techniques like correlation analysis and feature importance from tree-based models to select the most relevant features.
- Model Selection:
- Tried various models, including logistic regression, decision trees, random forests, and gradient boosting.
- Used k-fold cross-validation to evaluate model performance and avoid overfitting.
- Model Training and Evaluation:
- Trained the selected model (Gradient Boosting) on the training data.
- Evaluated the model using metrics like precision, recall, F1-score, and ROC-AUC.
- Hyperparameter Tuning:
- Used grid search and random search to find the best hyperparameters for the model.
- Deployment:
- Deployed the model into a production environment using a cloud-based platform.
- Set up a pipeline to regularly update the model with new data.
- Monitoring and Maintenance:
- Monitored the model’s performance over time and retrained it periodically to ensure accuracy.
Impact:
- The model achieved an F1-score of 0.85, significantly improving the ability to predict churn.
- The company implemented targeted retention strategies based on the model’s predictions, reducing churn by 20%.
- Increased customer satisfaction and loyalty, leading to higher revenue and growth.
This project not only solved a critical business problem but also demonstrated the value of data-driven decision-making in improving customer retention and business performance.
- Introducing OpenAI’s Operator: Your AI Assistant for Everyday Tasks with 100% ease
- Must Read New 15 Data Science Interview Questions Asked at Amazon with Answers for 100% success
- The Top 5 Free ChatGPT Alternatives You Can Try Today
- Top 20 Free LLM Interview Questions and Answers for 2025
- Top 21 Must Read Accenture Gen AI Interview Question